Breaking The Barrier: Swift's PDF Printing Quandary And The Solution You Need
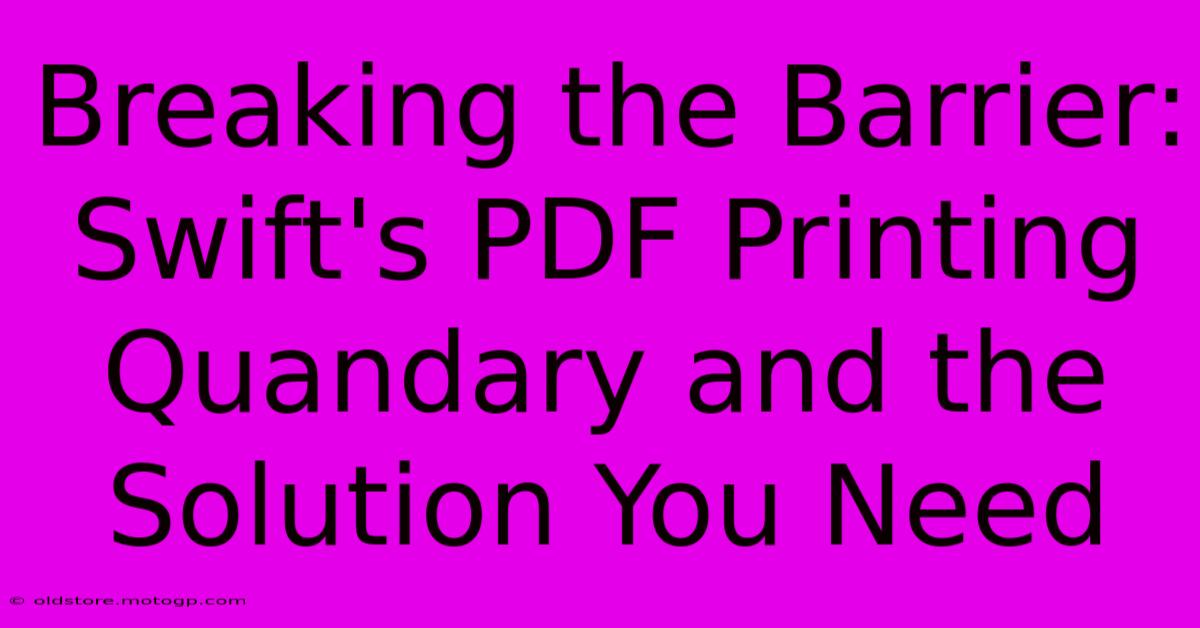
Table of Contents
Breaking the Barrier: Swift's PDF Printing Quandary and the Solution You Need
Printing PDFs from a Swift application might seem straightforward, but it's a surprisingly common source of frustration for developers. The lack of a built-in, universally reliable solution within the Swift ecosystem often leads to unexpected complications and inconsistent results across different devices and iOS versions. This article will delve into the challenges of PDF printing in Swift, exploring common pitfalls and presenting a robust, reliable solution to overcome them.
The Challenges of PDF Printing in Swift
Swift's approach to PDF handling isn't always intuitive. While frameworks exist for creating and manipulating PDFs, directly printing them often involves navigating a complex landscape of system APIs and potential compatibility issues. Here are some key challenges:
1. Inconsistent System Behavior:
Apple's printing system, while powerful, isn't always predictable. The behavior can vary depending on the connected printer, the iOS version, and even the user's system settings. This inconsistency makes creating a truly reliable printing solution a significant undertaking.
2. UI complexities:
Implementing a user-friendly printing interface that respects iOS design guidelines and provides granular control over print settings (like page ranges, margins, and paper size) can be complex. Developers need to carefully manage the user experience to ensure a smooth and intuitive printing process.
3. Handling Different PDF Libraries:
Various third-party libraries exist for PDF generation and manipulation in Swift. Choosing the right library and integrating it seamlessly into your application requires careful consideration of factors like performance, features, and licensing. Each library may have its own quirks and limitations when it comes to printing.
4. Debugging Difficulties:
Troubleshooting printing issues can be incredibly time-consuming. Errors often manifest subtly, making it difficult to pinpoint the root cause. Log messages and error handling are crucial but may not always provide sufficient information to diagnose the problem effectively.
A Robust Solution: Leveraging UIPrintInteractionController
While there isn't a single magic bullet, the UIPrintInteractionController
offers a relatively reliable approach to tackling the PDF printing challenge. This built-in iOS class provides a standardized interface for interacting with the system's printing capabilities. Here's a breakdown of how to leverage its power:
Step-by-Step Implementation:
-
Prepare your PDF Data: Ensure your PDF data is accessible (e.g., from a file, a URL, or generated in-app).
-
Create a
UIPrintInfo
Object: This object defines essential printing parameters like the printer job name and output type. -
Initialize
UIPrintInteractionController
: Create an instance ofUIPrintInteractionController
and assign theprintInfo
object. -
Set the Print Data: Assign your PDF data (usually as a
NSData
object) to theUIPrintInteractionController
'sprintingItem
property. -
Present the Print Interface: Use the
present(animated:completionHandler:)
method to display the system's standard print interface. This allows users to select a printer, choose print options, and initiate the printing process.
Code Example Snippet (Illustrative):
// Assuming 'pdfData' is your PDF data as NSData
let printInfo = UIPrintInfo(dictionary: nil)
printInfo.jobName = "My PDF Document"
let printInteractionController = UIPrintInteractionController.shared
printInteractionController.printInfo = printInfo
printInteractionController.printingItem = pdfData
printInteractionController.present(animated: true, completionHandler: { (completed, error) in
if let error = error {
print("Printing failed: \(error)")
} else if completed {
print("Printing successful!")
}
})
Best Practices for Success
-
Thorough Testing: Test your printing functionality extensively across different devices, iOS versions, and printers.
-
Error Handling: Implement robust error handling to gracefully manage potential printing failures.
-
User Feedback: Provide clear feedback to the user, indicating the progress and outcome of the printing process.
-
Consider Alternatives: If
UIPrintInteractionController
proves insufficient for your specific needs, explore third-party libraries that offer more advanced features for PDF generation and printing.
By carefully addressing the inherent challenges and leveraging the power of UIPrintInteractionController
along with robust error handling and testing, you can overcome the PDF printing quandary in Swift and create a reliable and user-friendly printing experience within your applications. This approach provides a solid foundation for handling PDF printing, allowing your application to seamlessly integrate with the system's printing capabilities.
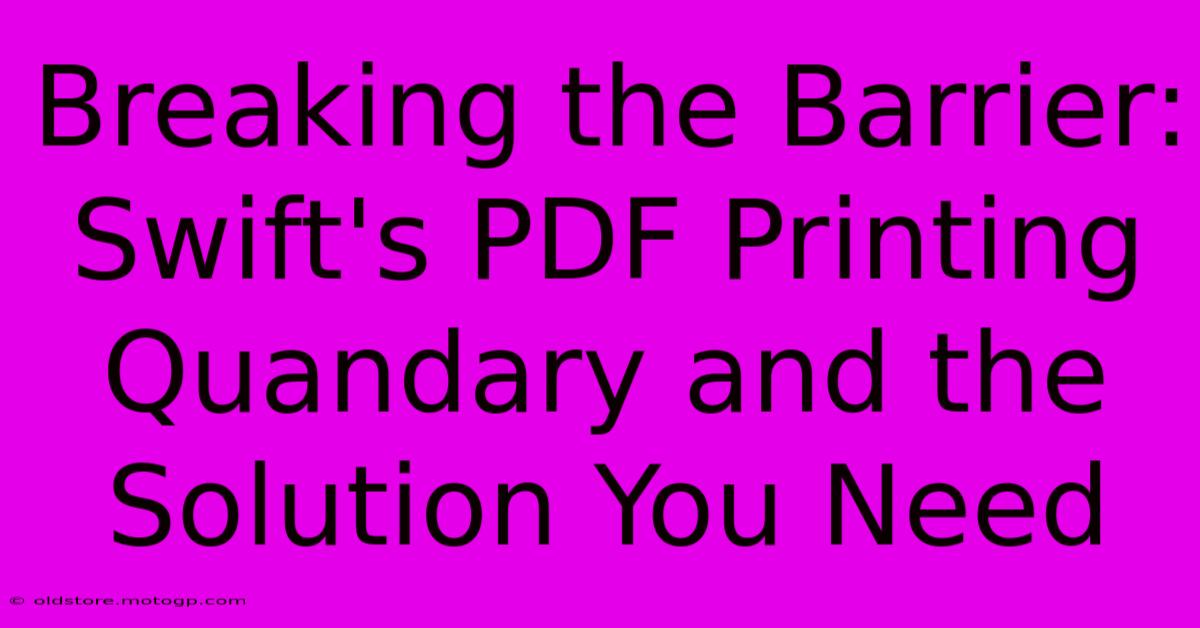
Thank you for visiting our website wich cover about Breaking The Barrier: Swift's PDF Printing Quandary And The Solution You Need. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Apologizing Amidst The Chaos We Re Not Hiding We Re Facing The Issue
Feb 06, 2025
-
The Mushroom Kingdoms Hidden Gem Discover The True Meaning Behind The Super Mario Logo
Feb 06, 2025
-
Dice Your Way To Perfection Transform Nails Into Fantasy Masterpieces
Feb 06, 2025
-
The Hidden Hues Decoding The Language Of Baby Breath Colors
Feb 06, 2025
-
Hex Ceptional Discovery Uncovering The Hidden Alchemy Of Apple Sunglow
Feb 06, 2025