Enhance Your Documents With Precision: Mastering The VBA Redaction Function
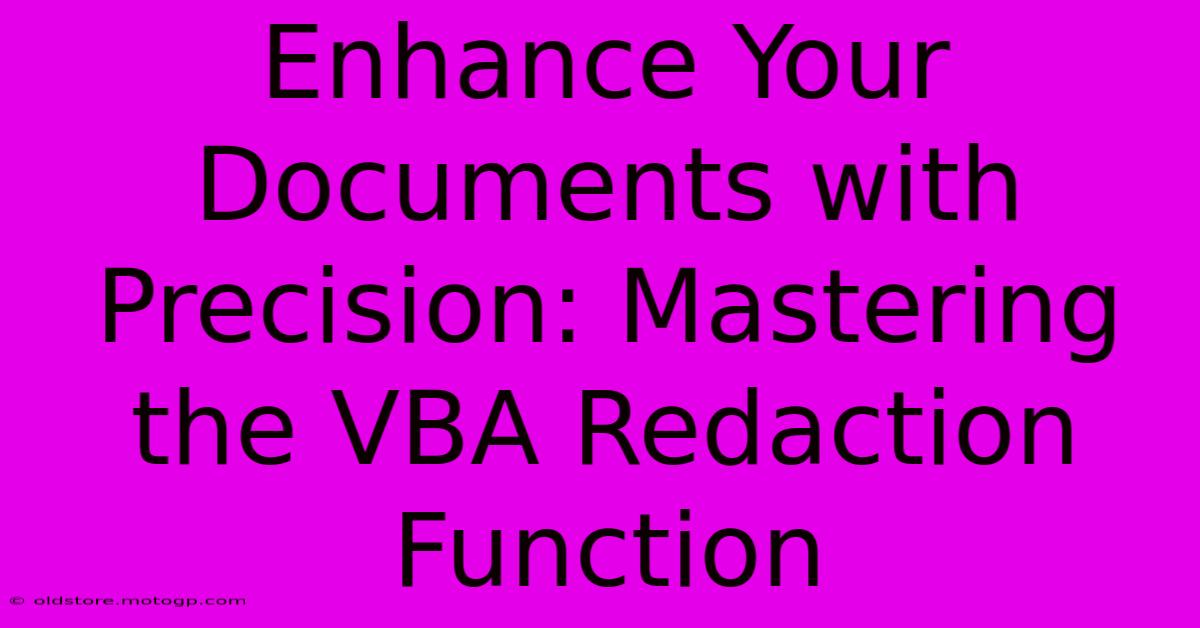
Table of Contents
Enhance Your Documents with Precision: Mastering the VBA Redaction Function
In today's data-driven world, protecting sensitive information is paramount. Whether you're handling legal documents, financial reports, or internal memos, ensuring confidentiality is crucial. While manual redaction is tedious and prone to error, VBA (Visual Basic for Applications) offers a powerful solution: the ability to automate redaction with precision and efficiency. This comprehensive guide will empower you to master the VBA redaction function, significantly streamlining your workflow and bolstering your data security.
Understanding the Need for Automated Redaction
Manually redacting documents involves painstakingly highlighting or blacking out sensitive data. This process is time-consuming, repetitive, and leaves room for human error. Omissions or incomplete redactions can lead to serious breaches of confidentiality and potentially severe legal consequences. Automated redaction using VBA eliminates these risks, providing a faster, more accurate, and reliable solution.
The Advantages of VBA Redaction
- Speed and Efficiency: VBA significantly reduces the time required for redaction, allowing you to process large volumes of documents quickly.
- Accuracy and Consistency: Automated redaction minimizes human error, ensuring consistent application of redaction across all documents.
- Improved Security: Reduced human intervention translates to a lower risk of accidental disclosure of sensitive information.
- Scalability: VBA redaction can easily handle large document sets, adapting to increasing data volumes.
- Customization: You can tailor the VBA code to meet your specific redaction needs, targeting particular keywords, patterns, or data types.
Building Your VBA Redaction Function
Let's delve into the practical aspects of creating a VBA redaction function. While the specific implementation will depend on your exact needs and the structure of your documents, the following example provides a strong foundation. This code assumes you're working within Microsoft Word.
Sub RedactDocument()
Dim objWord As Object, objDoc As Object
Dim strFind As String, strReplace As String
'Specify the text to be redacted. You can modify this as needed.
strFind = "Confidential Information"
'Specify the replacement text (usually a blank space or a series of asterisks).
strReplace = "****************" 'or "" for a blank space
'Create a Word application object
Set objWord = CreateObject("Word.Application")
'Open your document. Replace "C:\path\to\your\document.docx" with your file path
Set objDoc = objWord.Documents.Open("C:\path\to\your\document.docx")
'Perform the redaction using the Replace method. The option wdReplaceAll ensures all instances are replaced.
objDoc.Content.Find.Execute FindText:=strFind, ReplaceWith:=strReplace, Replace:=wdReplaceAll
'Save and close the document.
objDoc.Save
objDoc.Close
'Quit the Word application.
objWord.Quit
'Clean up objects. Very important!
Set objDoc = Nothing
Set objWord = Nothing
MsgBox "Redaction complete!"
End Sub
Refining Your VBA Redaction: Advanced Techniques
This basic example provides a starting point. For more sophisticated redaction needs, consider these enhancements:
- Regular Expressions: Employ regular expressions to target more complex patterns within your text, improving the precision of your redaction.
- Wildcard Characters: Utilize wildcard characters to match variations of keywords or data fields.
- Multiple Redaction Terms: Adapt the code to handle a list of words or phrases to be redacted.
- User Input: Prompt the user for input to specify the redaction terms dynamically.
- Redaction Formatting: Instead of simply replacing text, you can format the redacted text with specific colors, fonts, or shading for improved visual clarity.
Implementing and Optimizing Your VBA Redaction Solution
Once you have created your VBA redaction function, remember to test it thoroughly on sample documents before applying it to sensitive data. Start with a small subset of your documents to ensure the code performs as expected and doesn't introduce any unintended consequences. Regularly review and update your VBA code to maintain its accuracy and efficiency as your redaction requirements evolve.
Best Practices for Secure Redaction
- Version Control: Maintain versions of your VBA code to track changes and facilitate rollback if necessary.
- Secure Storage: Store your VBA code securely to prevent unauthorized access and modification.
- Testing and Validation: Thorough testing is vital to ensure the reliability and accuracy of your redaction process.
- User Training: Train users on proper document handling and redaction procedures to minimize the risk of human error.
By mastering the VBA redaction function, you can transform your approach to data protection, improving efficiency, accuracy, and overall security. Embrace this powerful tool and safeguard your sensitive information with confidence. Remember always to prioritize data security and follow best practices when handling confidential information.
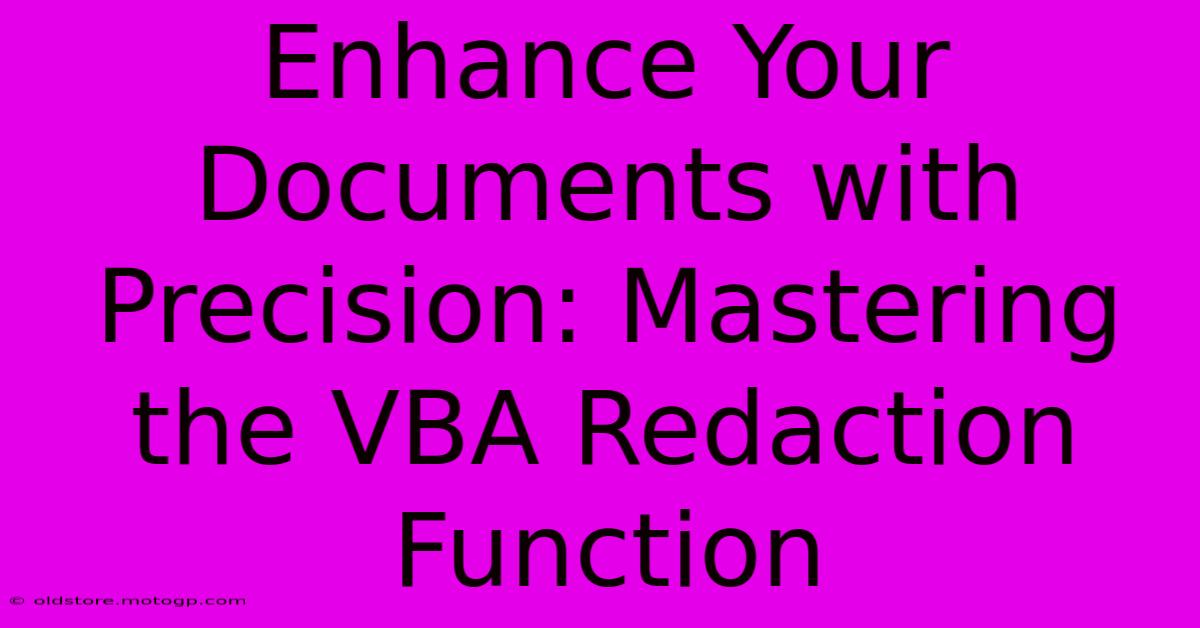
Thank you for visiting our website wich cover about Enhance Your Documents With Precision: Mastering The VBA Redaction Function. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Livry Gargan Mort Par Arme Blanche
Feb 05, 2025
-
El Extravagante Tocado De Los Grammy
Feb 05, 2025
-
College Athletes Cashing In The Stunning Salaries Of Nil Superstars
Feb 05, 2025
-
Oerebro Skyderi 10 Draebte
Feb 05, 2025
-
Temperatures Glaciales Neige En Plaine
Feb 05, 2025