Insider Tips: Optimizing VBA Word Redactions For Lightning-Fast Performance
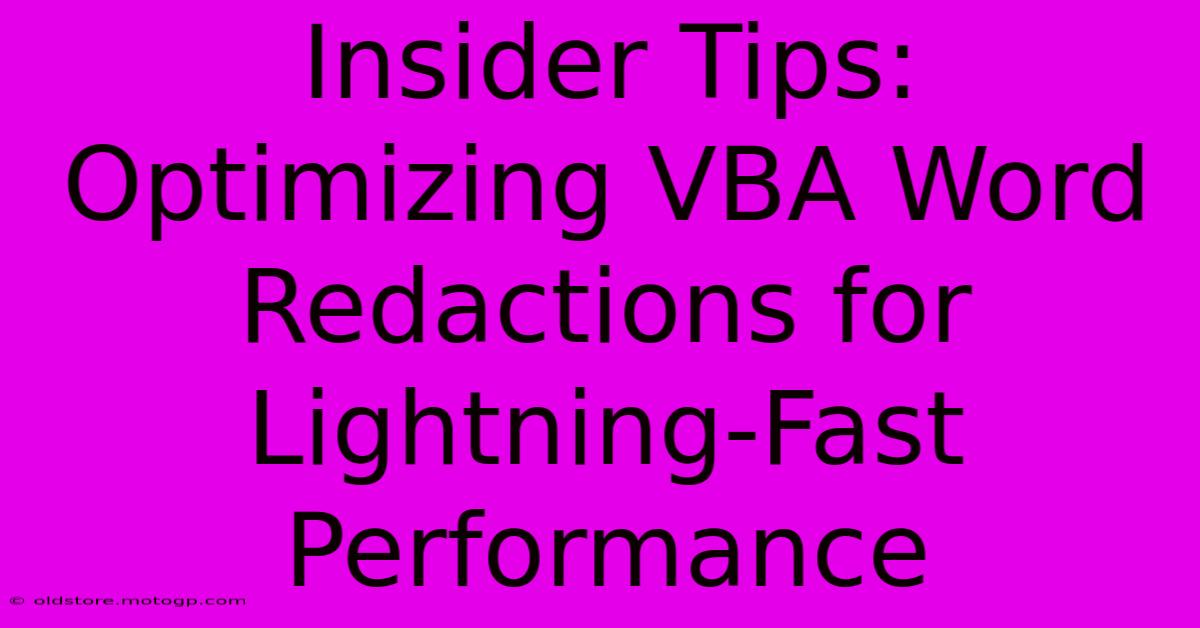
Table of Contents
Insider Tips: Optimizing VBA Word Redactions for Lightning-Fast Performance
Redacting sensitive information in Word documents is a crucial task, especially in legal and corporate settings. Manually redacting large documents is tedious and prone to errors. Visual Basic for Applications (VBA) offers a powerful solution, automating the process and significantly boosting efficiency. However, poorly written VBA code can lead to sluggish performance, especially with large files. This article reveals insider tips to optimize your VBA Word redaction macros for lightning-fast performance.
Understanding Performance Bottlenecks in VBA Redaction
Before diving into optimization techniques, let's identify common performance bottlenecks in VBA Word redaction macros:
1. Inefficient Selection and Replacement Methods:
Using Selection.Find
and Selection.Replace
repeatedly within loops can be incredibly slow, particularly with extensive documents. Word's object model isn't designed for rapid iterative changes on large scales.
2. Unnecessary Object Creation:
Creating and destroying objects within loops adds overhead. Reusing objects wherever possible reduces this burden.
3. Lack of Error Handling:
Unexpected errors can halt your macro. Robust error handling ensures graceful termination and prevents crashes, improving overall speed and reliability.
4. Screen Updates:
Continuously updating the screen during the redaction process slows down execution. Disabling screen updates while processing and re-enabling them at the end improves speed significantly.
Optimizing Your VBA Redaction Macros: Practical Strategies
Let's delve into specific techniques to optimize your VBA code for speed:
1. Leverage the Range
Object for Bulk Operations:
Instead of using Selection
, work directly with the Range
object. You can select and redact large sections of text simultaneously, dramatically improving performance. This avoids the constant recalculation and screen updates associated with using Selection
.
Sub FastRedaction(findText As String, redactionString As String)
Dim rng As Range
With ActiveDocument.Content.Find
.Text = findText
.Replacement.Text = redactionString
.Execute Replace:=wdReplaceAll
End With
End Sub
This code directly operates on the entire document's content, replacing all instances at once. This is far faster than iterating through each instance.
2. Employ the wdReplaceAll
Option:
The wdReplaceAll
option within the Find.Execute
method performs all replacements in a single operation, providing a substantial performance boost over iterating through each find and replace individually.
3. Minimize Object Creation:
Declare objects outside loops and reuse them. Creating new objects repeatedly within a loop consumes significant resources.
Sub EfficientLooping()
Dim doc As Document
Set doc = ActiveDocument
' ...your code using doc...
End Sub
4. Disable Screen Updating:
Temporarily disabling screen updates while the redaction process is running significantly speeds up execution. Re-enable it after the process completes to display the results.
Application.ScreenUpdating = False
' ... your redaction code here ...
Application.ScreenUpdating = True
5. Implement Robust Error Handling:
Include error handling using On Error Resume Next
or structured exception handling (On Error GoTo
) to gracefully handle unexpected situations. This prevents crashes and ensures the macro continues processing as much as possible.
On Error Resume Next
' ... your redaction code ...
If Err.Number <> 0 Then
MsgBox "An error occurred: " & Err.Description
End If
6. Optimize Redaction Method:
Consider using other methods to achieve the redaction. Instead of replacing text with a redaction character, you might explore techniques involving manipulating the document's XML structure for more efficient large-scale operations. This advanced approach offers the most significant performance gains.
Conclusion: Achieving Lightning-Fast Redaction with Optimized VBA
By implementing these optimization techniques, you can significantly enhance the performance of your VBA Word redaction macros. Moving beyond simply replacing text with a redaction character and instead exploring direct manipulation of Word's underlying structure can yield the most remarkable improvements. Remember, careful planning and code optimization are key to achieving lightning-fast performance when processing large documents. Experiment with different approaches and find the optimal balance between readability and speed for your specific needs.
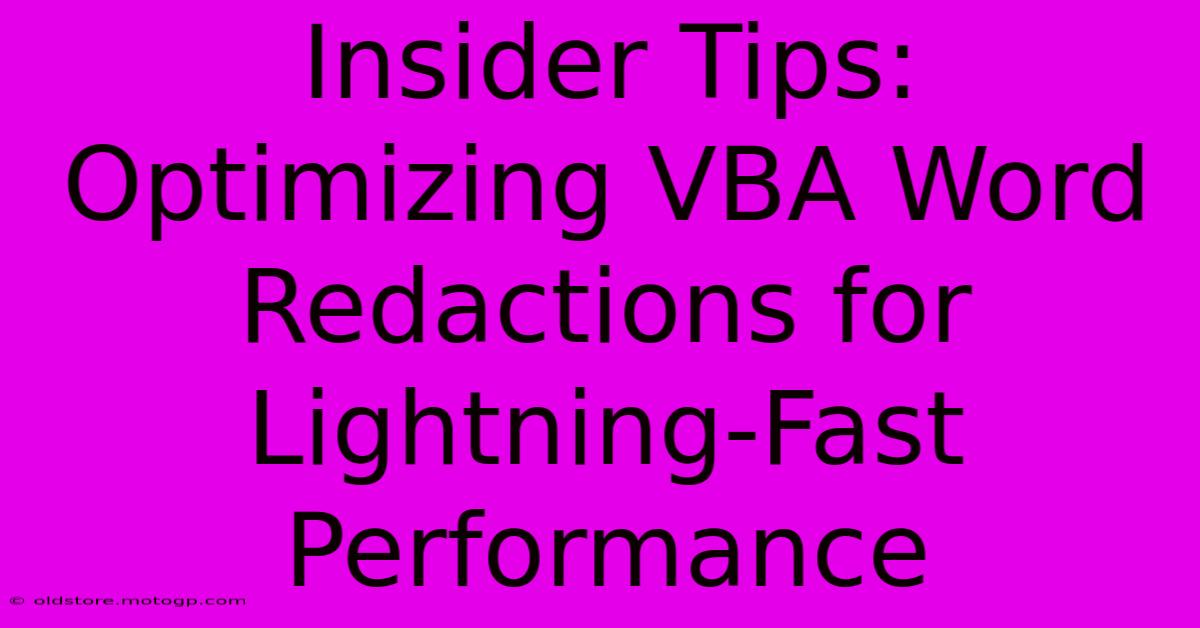
Thank you for visiting our website wich cover about Insider Tips: Optimizing VBA Word Redactions For Lightning-Fast Performance. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Any Nationality Us Deportees To El Salvador
Feb 05, 2025
-
Gold Plated Jewels The Ultimate Guide To Affordable Luxury And Radiant Adornment
Feb 05, 2025
-
Malmoebegravning Morduppmaning Mot 15 Aring
Feb 05, 2025
-
Master The Art Of Softness And Serenity The Ultimate Guide To Soft Ballet Pink Hex Code
Feb 05, 2025
-
Master The Art The Essential Elements Of A Winning Ux Design Portfolio
Feb 05, 2025