Replace Characters In VBA: The Ultimate VBA Spellbook
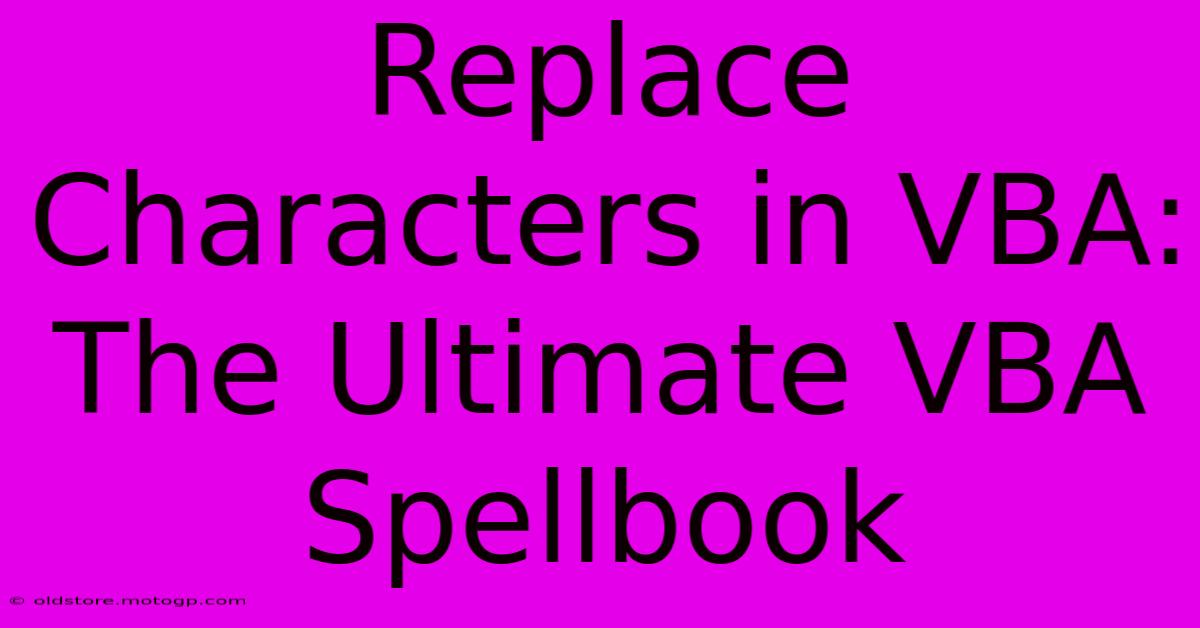
Table of Contents
Replace Characters in VBA: The Ultimate VBA Spellbook
So, you're working with VBA (Visual Basic for Applications) and need to replace characters within strings? You've come to the right place! This comprehensive guide will equip you with the magical spells – or rather, VBA code snippets – to conquer any character replacement challenge. We'll cover various scenarios, from simple swaps to complex manipulations, transforming your VBA projects from mundane to magnificent.
Understanding the Replace Function
The heart of character replacement in VBA lies within the Replace
function. It's a powerful tool that allows you to find and replace specific instances of a character or substring within a string. Let's explore its syntax:
Replace(string, find, replace, [start], [count], [compare])
- string: The original string where you'll perform the replacement.
- find: The character or substring you want to find and replace.
- replace: The character or substring you want to use as a replacement.
- start: (Optional) The position within the string where you want to start searching. Defaults to 1 (the beginning).
- count: (Optional) The number of occurrences to replace. Defaults to replacing all occurrences.
- compare: (Optional) Specifies the type of comparison (binary, text, or database). Defaults to binary.
Simple Character Replacement
Let's start with a basic example: replacing all occurrences of "a" with "A" in a string.
Sub SimpleReplace()
Dim myString As String
myString = "This is a sample string with lowercase a's."
myString = Replace(myString, "a", "A")
MsgBox myString ' Displays: "This is A sAmple string with lowercase A's."
End Sub
This code snippet demonstrates the most straightforward use of the Replace
function. It's clean, efficient, and perfect for simple replacements.
Advanced Techniques: Mastering the Spellbook
While the basic Replace
function handles many tasks, VBA offers more sophisticated approaches for complex character manipulation.
Replacing Multiple Characters Simultaneously
What if you need to replace multiple characters at once? Nested Replace
functions are your solution.
Sub MultipleReplace()
Dim myString As String
myString = "This string contains a, e, i, o, u vowels."
myString = Replace(Replace(Replace(Replace(Replace(myString, "a", "A"), "e", "E"), "i", "I"), "o", "O"), "u", "U")
MsgBox myString ' Displays: "ThIs strIng contAIns A, E, I, O, U vowels."
End Sub
While functional, nesting Replace
can become unwieldy for many replacements. A more elegant approach involves using regular expressions (Regex), which we'll discuss later.
Case-Insensitive Replacement
By default, Replace
is case-sensitive. To perform a case-insensitive replacement, you'll need to employ the StrComp
function with the vbTextCompare
option.
Sub CaseInsensitiveReplace()
Dim myString As String
myString = "This string has Mixed Case."
If StrComp(Mid(myString, 1, 1), "t", vbTextCompare) = 0 Then
myString = Replace(myString, "t", "T")
End If
MsgBox myString 'Displays a string with the first 't' replaced with 'T'
End Sub
This example demonstrates replacing only the first occurrence of "t" with "T" in a case-insensitive manner. You can expand this to replace all instances using loops and InStr
.
Utilizing Regular Expressions (Regex)
For intricate character manipulations, regular expressions offer unparalleled power. VBA doesn't have built-in Regex support, but you can leverage the VBScript.RegExp
object.
Sub RegexReplace()
Dim objRegExp As Object, myString As String
Set objRegExp = CreateObject("VBScript.RegExp")
myString = "This string has 123 numbers and some symbols like @#$%^&*()_+=-`~[]\{}|;':\",./<>?"
With objRegExp
.Global = True ' Replace all occurrences
.Pattern = "[^a-zA-Z0-9\s]" ' Matches non-alphanumeric characters and spaces
myString = .Replace(myString, "") 'Replaces all matched characters with empty string
End With
MsgBox myString ' Displays: "This string has 123 numbers and some symbols"
End Sub
This example removes all non-alphanumeric characters and spaces from a string. Regex opens up a world of possibilities for complex pattern matching and replacement.
Conclusion: Mastering the Art of Character Replacement
This guide has provided you with a powerful arsenal of VBA techniques for character replacement. From simple swaps using the Replace
function to more advanced methods involving nested Replace
, case-insensitive comparisons, and the potent capabilities of regular expressions, you now possess the tools to handle any character manipulation challenge. Remember to choose the method best suited to your specific needs for optimal efficiency and clarity. Happy coding!
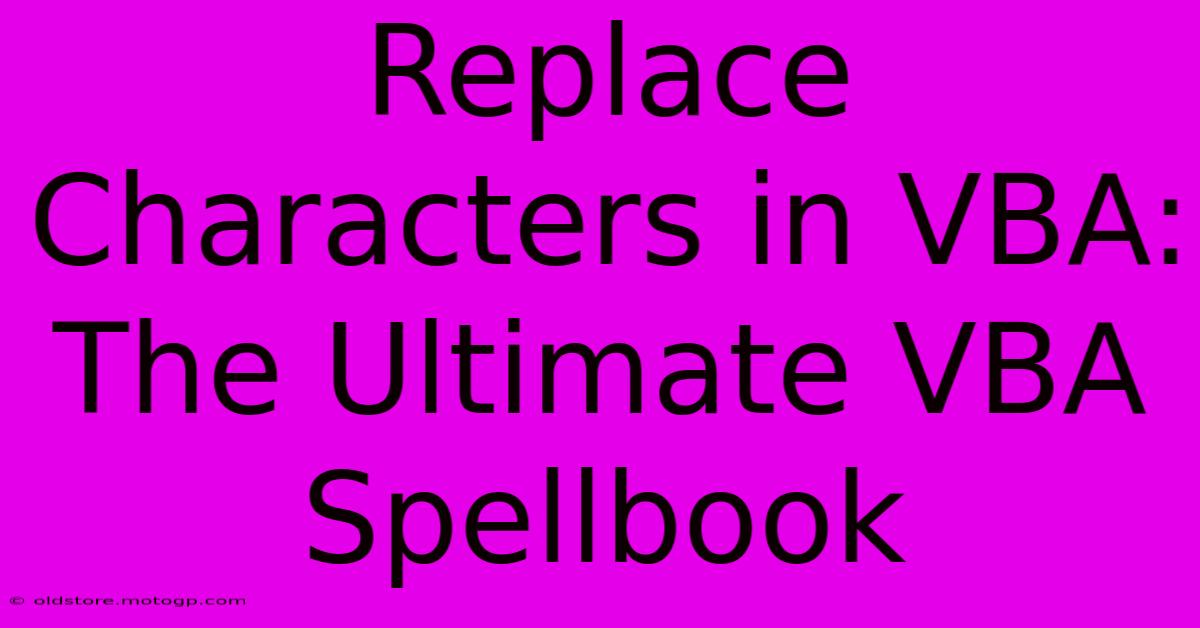
Thank you for visiting our website wich cover about Replace Characters In VBA: The Ultimate VBA Spellbook. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Elevate Your Fishing To Quantum Levels Introducing The Kvd Baitcast Reel
Mar 13, 2025
-
The Toblerone Bears Epic Quest A Journey Of Brand Identity
Mar 13, 2025
-
Finding Refuge In The Prince Of Peace How Christianity Brings Relief To Troubled Souls
Mar 13, 2025
-
Monstrous Makeovers Transforming Mu Frats From Dorm Rooms To Cursed Castles
Mar 13, 2025
-
From Anxiety To Assurance The Bibles Guide To Emotional Freedom
Mar 13, 2025