The Encapsulation Enigma: Unraveling The Mysteries Of Class-Based Objects
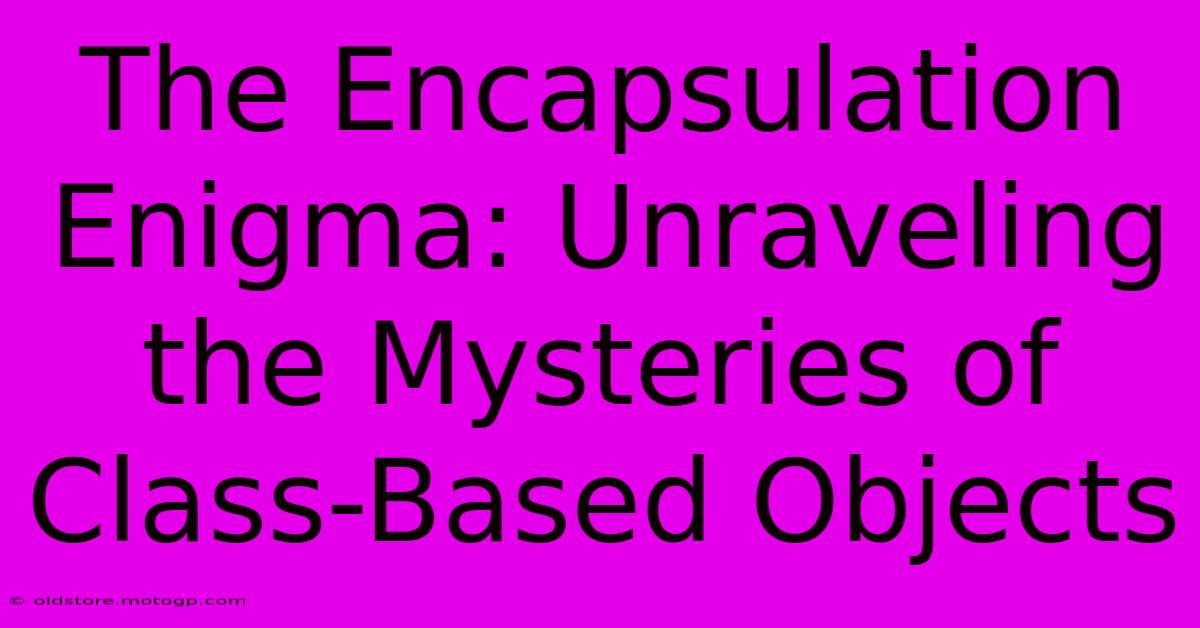
Table of Contents
The Encapsulation Enigma: Unraveling the Mysteries of Class-Based Objects
Encapsulation. The word itself sounds mysterious, doesn't it? But in the world of object-oriented programming (OOP), understanding encapsulation is crucial for writing clean, efficient, and maintainable code. This article delves into the heart of encapsulation, explaining its principles, benefits, and how it works within the context of class-based objects.
What is Encapsulation?
At its core, encapsulation is a fundamental OOP principle that bundles data (variables) and the methods (functions) that operate on that data within a single unit – a class. Think of it like a protective capsule: the data is safely enclosed, and access is controlled. This controlled access prevents unintended modifications and improves the overall integrity of your code.
Key Aspects of Encapsulation:
-
Data Hiding: Encapsulation hides the internal representation of an object from the outside world. This means that other parts of your program cannot directly access or manipulate the object's data. This protection is vital for data integrity and security.
-
Access Modifiers: Programming languages provide mechanisms (access modifiers like
public
,private
,protected
) to control the visibility and accessibility of class members.Private
members are only accessible within the class itself, enforcing strong encapsulation.Public
members can be accessed from anywhere, whileprotected
members offer a middle ground, accessible within the class and its subclasses. -
Abstraction: Encapsulation works hand-in-hand with abstraction. Abstraction simplifies complex systems by hiding unnecessary details and presenting only essential information to the user. By encapsulating data and methods, you abstract away the implementation details, allowing users to interact with the object without needing to know how it works internally.
The Benefits of Encapsulation
Why bother with encapsulation? The benefits are numerous and significant:
-
Data Security: By restricting direct access to data, encapsulation protects against accidental or malicious modification. This is particularly important in larger projects where multiple developers might be working on the same codebase.
-
Code Maintainability: Changes to the internal implementation of a class won't affect other parts of the program, as long as the public interface remains consistent. This makes maintenance and updates much easier and less error-prone.
-
Code Reusability: Encapsulated classes are highly reusable. You can easily integrate them into different parts of your project or even into other projects without worrying about conflicts or unintended side effects.
-
Improved Modularity: Encapsulation promotes modularity by breaking down complex systems into smaller, self-contained units. This makes the code easier to understand, debug, and test.
Implementing Encapsulation in Class-Based Objects
Let's illustrate encapsulation with a simple example using Python:
class Dog:
def __init__(self, name, breed):
self._name = name # Protected attribute
self._breed = breed # Protected attribute
def get_name(self):
return self._name
def get_breed(self):
return self._breed
def set_name(self, new_name):
self._name = new_name
def bark(self):
print("Woof!")
my_dog = Dog("Buddy", "Golden Retriever")
print(my_dog.get_name()) # Accessing data through methods
my_dog.set_name("Max") # Modifying data through methods
my_dog.bark()
#print(my_dog._name) #Direct access to _name is possible, but discouraged. It's a design choice, not a strict enforcement of privacy.
In this example, the _name
and _breed
attributes are protected (indicated by the underscore prefix – a Python convention). Direct access is discouraged; instead, we use getter (get_name
, get_breed
) and setter (set_name
) methods to interact with the data. This exemplifies proper encapsulation.
Beyond the Basics: Advanced Encapsulation Techniques
While the basic principles are straightforward, advanced techniques exist to further refine encapsulation. These include:
-
Design Patterns: Design patterns, such as the Factory and Singleton patterns, leverage encapsulation to create robust and flexible object structures.
-
Dependency Injection: This technique decouples classes by passing dependencies as parameters, rather than creating them internally, leading to better encapsulation and testability.
-
Immutability: Creating immutable objects prevents modification after creation, enhancing data integrity and simplifying concurrency management.
Conclusion: Mastering the Encapsulation Art
Encapsulation is more than just a programming concept; it's a design philosophy that promotes robust, maintainable, and reusable code. By carefully controlling access to data and methods, you build systems that are easier to understand, debug, and evolve. Mastering encapsulation is a critical step towards becoming a proficient object-oriented programmer. Embrace the enigma, and unlock the power of encapsulated class-based objects!
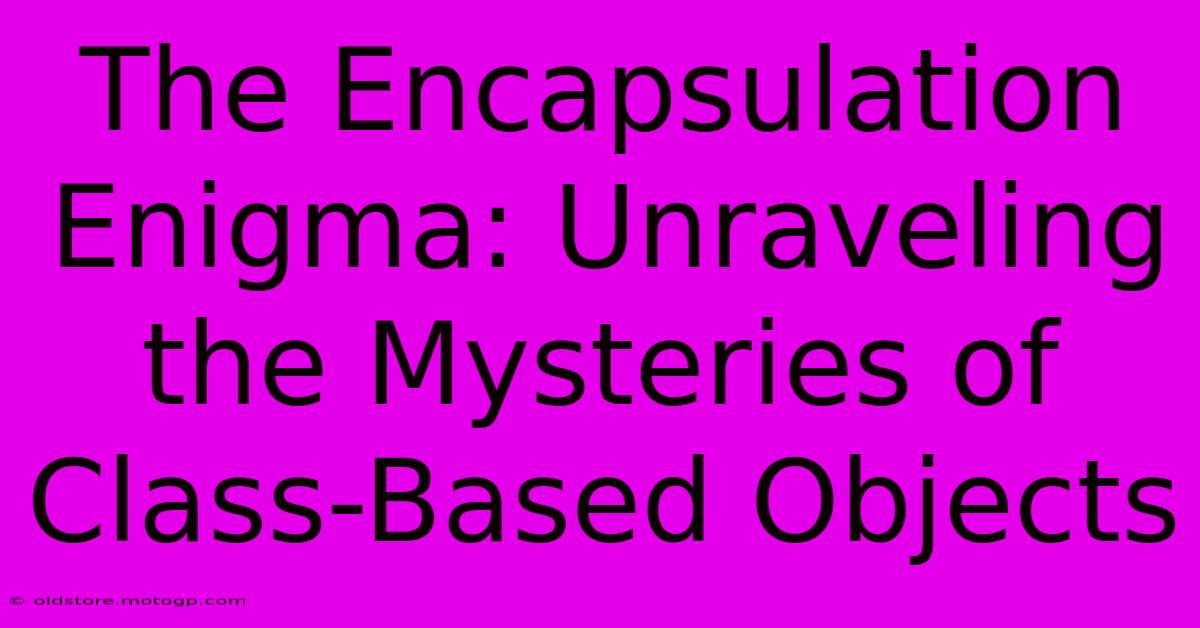
Thank you for visiting our website wich cover about The Encapsulation Enigma: Unraveling The Mysteries Of Class-Based Objects. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Exclusive Peek The Secret Garden Oasis At 7500 Grace Drive
Mar 10, 2025
-
Immerse Yourself In Magic Uncover Pixars Most Captivating And Award Winning Films
Mar 10, 2025
-
Quantum Projection The Secret Weapon For Solving Unsolvable Problems
Mar 10, 2025
-
Experience The Ultimate Waterfront Getaway At 4445 Corporation Lane Virginia Beach
Mar 10, 2025
-
Turquoise Tides Emerald Cliffs Paddle Into The Aquamarine Wonders Of Julys Shores
Mar 10, 2025