The React Component Showdown: Class Vs. Functional - Which Will Reign Supreme?
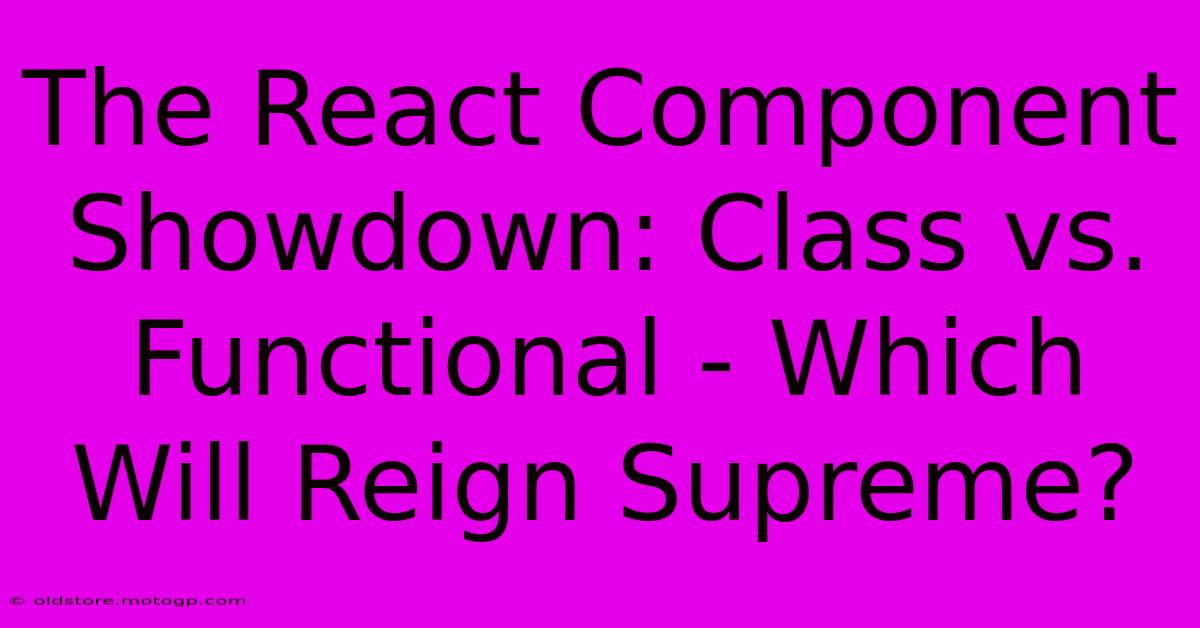
Table of Contents
The React Component Showdown: Class vs. Functional - Which Will Reign Supreme?
React, the JavaScript library for building user interfaces, offers two primary ways to create components: class components and functional components. For years, class components held sway, but with the advent of hooks, functional components have surged in popularity. This article delves into the strengths and weaknesses of each, helping you determine which approach best suits your project.
The Rise of Functional Components with Hooks
Before React Hooks, functional components were simple, stateless functions. Their primary role was to display data passed as props. However, the introduction of Hooks fundamentally changed the game. Hooks like useState
, useEffect
, useContext
, and others brought state management and lifecycle methods directly into functional components. This eliminated the need for complex class structures and significantly simplified component development.
Advantages of Functional Components with Hooks:
- Conciseness and Readability: Functional components are often shorter and easier to read than their class component counterparts, leading to improved code maintainability. This is particularly noticeable in more complex components.
- Improved Reusability: Hooks promote code reuse by encapsulating specific logic into reusable functions. This leads to more modular and maintainable code.
- Simpler Testing: Testing functional components is generally easier because they lack the complexities associated with class component lifecycle methods.
- Better Performance (in some cases): Functional components can sometimes offer slightly better performance due to their simpler structure, although the difference is often negligible in most applications.
Example: A simple counter with a functional component and useState
hook:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
You clicked {count} times
);
}
export default Counter;
The Legacy of Class Components
Class components were the original way to create components in React. They utilize ES6 classes and provide access to lifecycle methods like componentDidMount
, componentDidUpdate
, and componentWillUnmount
. While less popular now, they still have a place in certain scenarios.
Advantages of Class Components (niche cases):
- Familiarity (for experienced developers): Developers familiar with object-oriented programming may find class components more intuitive.
- Direct Access to Lifecycle Methods (though Hooks now provide equivalents): While Hooks offer similar functionality, some developers might find the explicitness of class lifecycle methods beneficial. This is becoming increasingly less relevant.
Example: The same counter implemented as a class component:
import React from 'react';
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
render() {
return (
You clicked {this.state.count} times
);
}
}
export default Counter;
Which Reigns Supreme? The Verdict
For the vast majority of use cases, functional components with Hooks are the clear winner. Their simplicity, readability, and improved features make them the preferred choice for new projects and for refactoring existing codebases. Class components are largely legacy code, and while they might still exist in some older projects, there's little reason to choose them for new development. Unless you have a compelling reason rooted in specific legacy code requirements or existing team expertise, stick with functional components and embrace the power of Hooks.
Beyond the Showdown: Best Practices
Regardless of which type of component you choose, adhering to best practices is crucial for maintaining a healthy and efficient codebase. This includes:
- Small, focused components: Keep your components concise and focused on a single task or feature.
- Meaningful component names: Use descriptive names that clearly indicate the purpose of each component.
- Consistent coding style: Follow a consistent coding style guide to ensure readability and maintainability.
- Proper state management: Choose appropriate state management techniques based on your application's complexity.
By following these guidelines, you can ensure that your React applications are efficient, maintainable, and scalable. The choice between class and functional components is largely a matter of choosing the best tool for the job, and in today's React ecosystem, that tool is almost certainly the functional component.
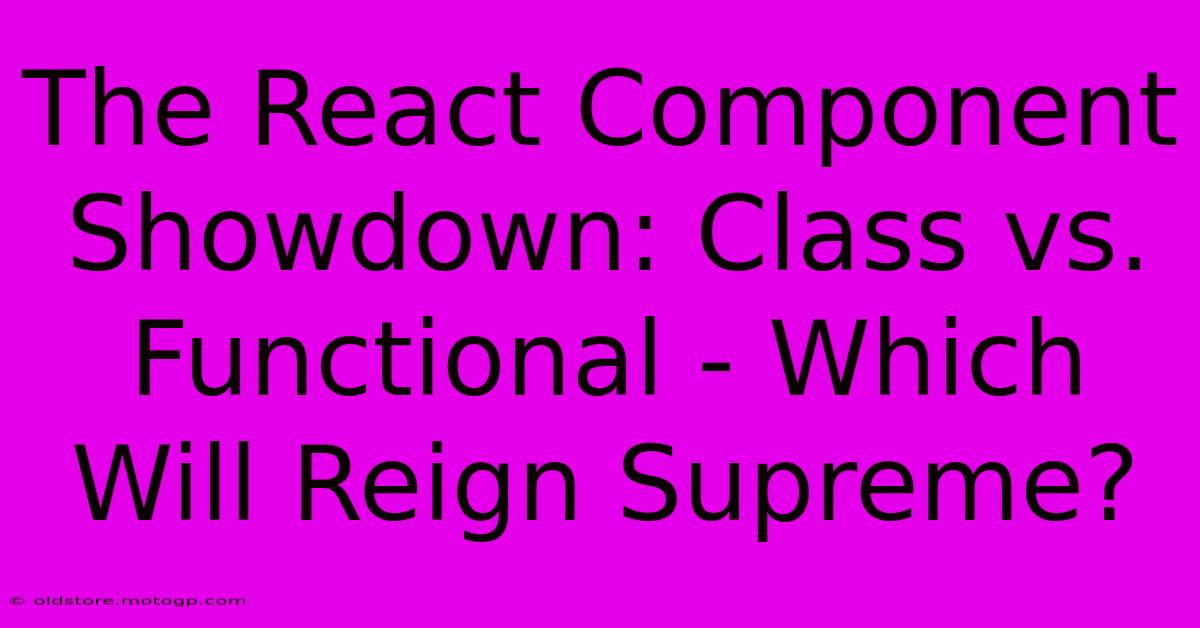
Thank you for visiting our website wich cover about The React Component Showdown: Class Vs. Functional - Which Will Reign Supreme?. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Step Back In Time With An Antique Wonder Discover The Charm Of The Gas Cook Stove
Mar 04, 2025
-
Unveiling The Quantum Toolkit Ultimate Gifts For The Architects Of Tomorrow
Mar 04, 2025
-
Forbidden Fruit Unveiled Taste The Ethereal Delight Of 478 Spiced Berry
Mar 04, 2025
-
The Solar Observatory Of Ancient Americans Sunwatch Indian Village Revealed
Mar 04, 2025
-
Dimensions Decoded Unlock The Secrets Of American Flag Measurements
Mar 04, 2025