The VBA Variable Revolution: Redefining Form Control Customization
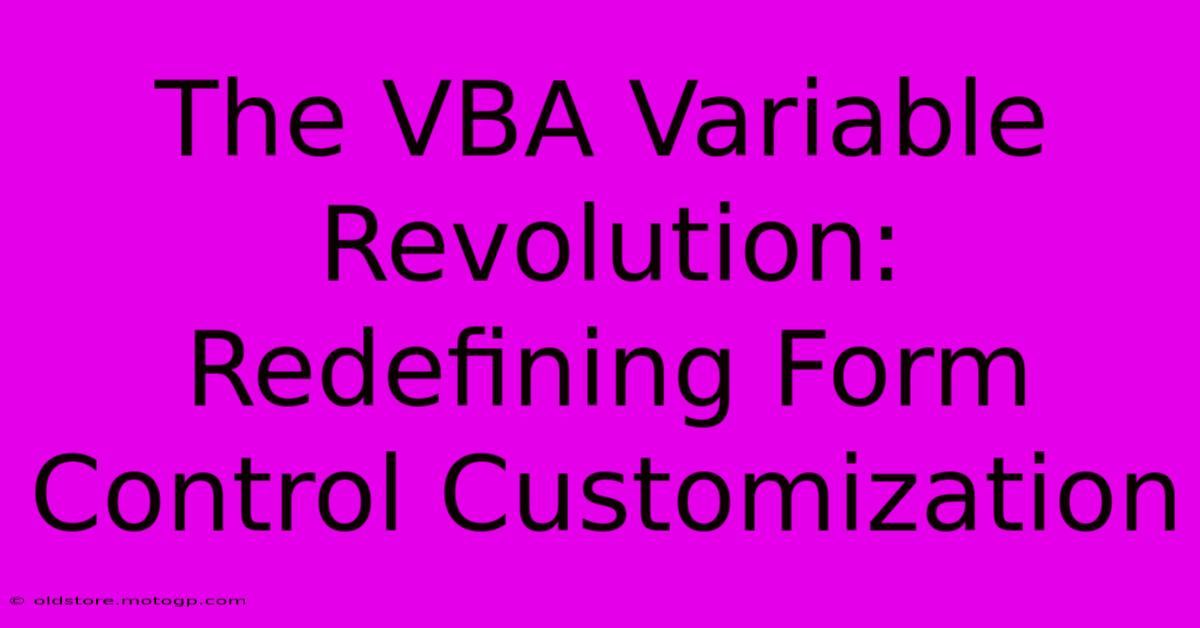
Table of Contents
The VBA Variable Revolution: Redefining Form Control Customization
Visual Basic for Applications (VBA) is a powerful tool that breathes life into Microsoft Office applications. While many users are familiar with its basic functionalities, the true potential of VBA lies in its ability to deeply customize form controls, unlocking a level of control previously unimaginable. This article explores how leveraging VBA variables revolutionizes form control customization, empowering you to create dynamic and responsive user interfaces.
Understanding the Power of VBA Variables in Form Control Customization
VBA variables are the cornerstone of dynamic form control manipulation. Unlike statically defined properties, variables allow you to store and manipulate data, enabling you to create forms that react to user input and changing conditions. This dynamic behavior vastly expands the possibilities of what you can achieve with your forms.
Why Variables are Essential:
- Flexibility: Variables allow you to change properties of form controls (like labels, captions, visibility, and enabled states) on-the-fly, based on user actions or other events.
- Reusability: Code becomes cleaner and more efficient by using variables to store frequently used values, reducing redundancy and improving maintainability.
- Dynamic Behavior: Create interactive forms that respond dynamically to user input, providing a more engaging and intuitive user experience.
- Data Management: Variables efficiently manage and process data entered into form controls, simplifying data handling and validation processes.
Practical Examples: Transforming Your Forms with VBA Variables
Let's illustrate the power of VBA variables with a few practical examples:
Example 1: Dynamically Updating Labels Based on User Input
Imagine a form with a textbox where the user enters a number. A label next to the textbox should display "Number Entered: [user input]". Using VBA variables, you can achieve this easily:
Private Sub TextBox1_Change()
Dim userNumber As Integer
userNumber = TextBox1.Value 'Store the user input in a variable
'Update the label with the value stored in the variable
Label1.Caption = "Number Entered: " & userNumber
End Sub
This simple code snippet demonstrates how a variable (userNumber
) stores the textbox value, then updates the label caption dynamically.
Example 2: Conditional Visibility of Form Controls
Let's say you want to show or hide a specific form control based on a checkbox selection. Here's how variables help:
Private Sub CheckBox1_Click()
Dim showControl As Boolean
showControl = CheckBox1.Value 'Store the checkbox state in a variable
'Control visibility based on the variable
If showControl Then
TextBox2.Visible = True
Else
TextBox2.Visible = False
End If
End Sub
The showControl
variable neatly manages the visibility of TextBox2
based on the checkbox's state.
Example 3: Enhanced Data Validation with Variables
Employing variables significantly improves data validation within your forms:
Private Sub CommandButton1_Click()
Dim inputValue As String
inputValue = TextBox1.Value
If IsNumeric(inputValue) Then
'Process numeric data
MsgBox "Valid input: " & inputValue
Else
'Handle invalid input
MsgBox "Invalid input. Please enter a number."
TextBox1.SetFocus
End If
End Sub
Here, inputValue
stores and validates user input before processing, preventing errors and enhancing the form's robustness.
Beyond the Basics: Advanced Techniques with VBA Variables
Beyond these basic examples, VBA variables unlock the door to complex form control customizations:
- Arrays of Variables: Manage multiple form controls efficiently using arrays.
- User Defined Types (UDTs): Structure related data for improved organization and code readability.
- Event Handling: React to various events (like mouse clicks, key presses) to create highly interactive forms.
Conclusion: Unleash the Power of Variable-Driven Form Control Customization
Mastering VBA variables is crucial for creating sophisticated and dynamic forms. The examples presented above represent just a glimpse into the vast potential of this approach. By incorporating these techniques into your VBA coding, you can significantly enhance the functionality, user experience, and overall effectiveness of your custom forms within Microsoft Office applications. Embrace the power of variables and transform your form control customization!
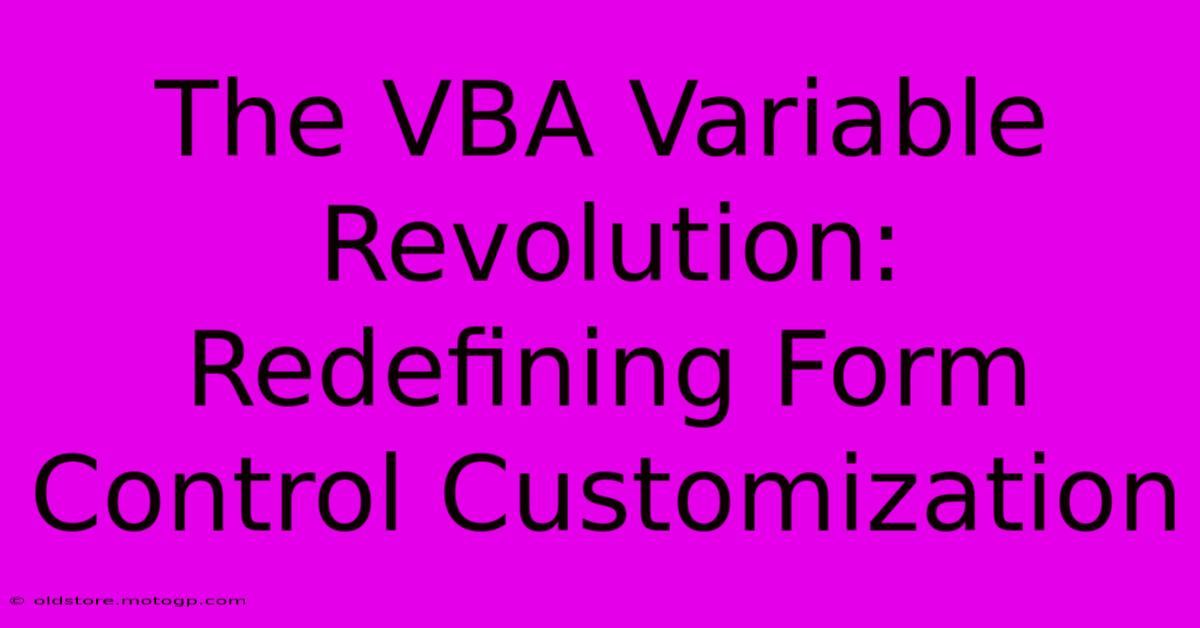
Thank you for visiting our website wich cover about The VBA Variable Revolution: Redefining Form Control Customization. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Discover The Power Of After School Activities Supercharge Your Childs Mind And Body
Feb 06, 2025
-
Unlock The Secret Hex How To Find The Code For Pms 291
Feb 06, 2025
-
Risparmia Tempo E Banda Comprimi Immagini Ad Alta Velocita Per Caricamenti Web Migliori
Feb 06, 2025
-
Breaking News Polyureas Game Changing Protection For Embassies
Feb 06, 2025
-
Automotive Coatings 101 The Ultimate Guide For Manufacturers
Feb 06, 2025